Server Events and Client Scripts
PHP Report Maker now supports server events and client scripts. You can create
your own functionality by writing your own customized server and client side
codes. The customized codes are stored in your projects so you can migrate
your project to other templates or future versions easily. This feature
reduces the need of template customization and create maximum possibilities
for you to customize and create more advanced features for your projects.
The steps to create the server events and client scripts are as follows:
After loading the database, the database objects (tables, views, custom
views and reports) will be shown in the left pane (the database pane).
Click on any table to go to the Field Setup Page and the Server
Events and Client Scripts page at any time.
Note: For simplicity, we use "table" in the following description to refer to any of database object in the project. A database object can be either a table, a view, a custom view or a report.
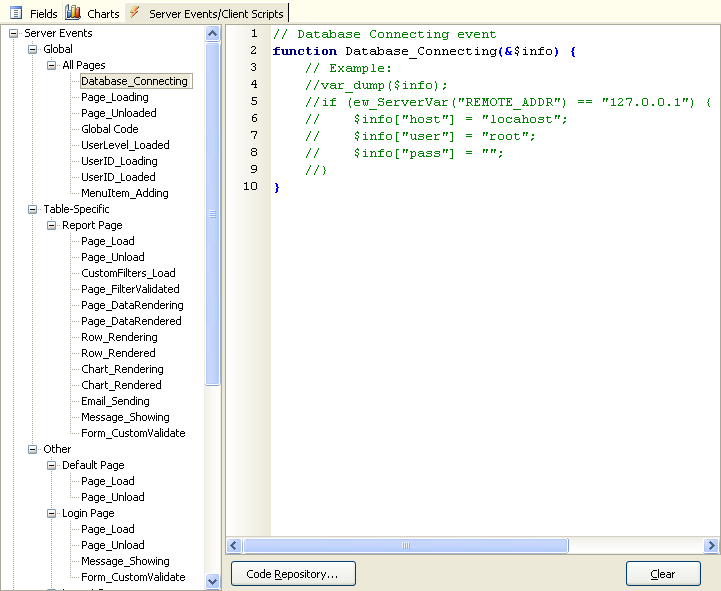
The treeview shows the available server events and client scripts that you can add to your project:
Server Events |
Server-side VBScript procedures |
Global |
The events are applicable to all PHP pages |
Table-Specific |
The set of events are table-specific, the events are applicable to the selected table only |
Other |
The events are applicable to some common pages in the project only |
Client Scripts |
Client-side JavaScript |
Global |
The JavaScript are included in all pages with header and footer |
Table-Specific |
The JavaScript are table-specific, they are included to pages for the selected table only |
Other |
The JavaScript are included in some common pages in the project only |
To add your custom scripts to the server events or client scripts, select
an item in the treeview, then enter your code in the editor.
The editor supports Find and Replace. Press Ctrl-F to open the Find dialog and press Ctrl-H to open the Replace dialog.
You can click the [Reset] button to discard the existing code and reload template code for the server event or client script.
Code Repository
PHP Report Maker now provides a Code Repository for easy reuse of your code across
projects and sharing with other users. Click the [Code Repository]
button to open it, categorized reusable code will be displayed:
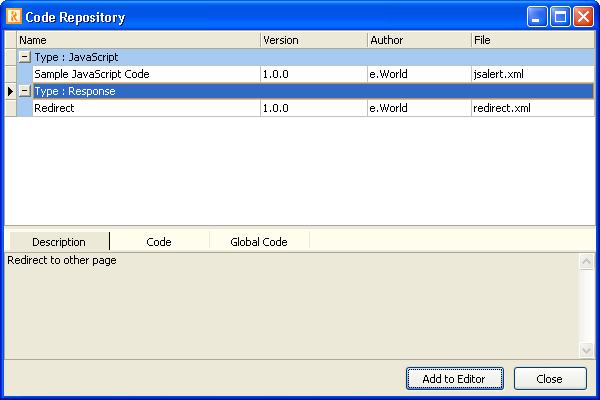
You can add the code to the editor by clicking the [Add to Editor] button, or by double-clicking the item, or simply drag the item to the editor. The reusable code is stored in XML files which reside in s subfolder name "code" under the installation folder. The format of the XML files is simple, there are 3 parts:
- description - description of the reusable code
- code - code to be inserted to editor
- globalcode - common code to be inserted to Global Code (see below), this code will only be inserted once into the project. For example , if your code is used several times in your project and your code calls a helper function, it is unnecessary to include the helper function several times. In this case you put your helper function in the globalcode section, then the function will only be included one time.
There are a few example files in the "code" folder, you can copy and modify for your own code and then save them under the "code" folder for reuse.
Server Events
In general, server events are fired in the following order:
Page_Load (Table-Specific/Other, a page class member) -> Row_* (Table-Specific, a table class member) -> Page_Unload (Table-Specific/Other, a page class member)
Available server events are:
Global -> All Pages |
Page_Head |
The code you entered in this event will be placed in the header.php before closing the <head> section. You can use this event to can add your code in head section.
Example
Include custom JavaScript and CSS styesheet.
ewrpt_AddClientScript("xxx.js"); // Add JavaScript
ewrpt_AddStylesheet("xxx.css"); // Add CSS stylesheet |
Database_Connecting |
This event will be fired by all PHP pages before connecting to the database. Note: This is a global function.
If MySQL/PostgreSQL, the argument is an array with the following keys: host, port, user, pass, db. If Access/MSSQL, the argument is the connection string for ADO. You can use this event to change the connection string (e.g. changing connection info with server, or even connect to other databases).
Example
// MySQL/PostgreSQL
function Database_Connecting(&$info) {
//var_dump($info);
if (ewrpt_CurrentUserIP() == "127.0.0.1") {
$info["host"] = "localhost";
$info["user"] = "root";
$info["pass"] = "";
}
} |
Database_Connected |
This event will be fired by all PHP pages after connecting to the database. Note: This is a global function.
The argument is the connection object, you can use it to execute your own statements.
Example
Call a stored procedure after connection.
function Database_Connected(&$conn) {
$conn->Execute("CALL MyStoredProcedure");
} |
Page_Loading |
This event will be called by all PHP pages at the beginning of the page. If the pages involves database connection, it will be called after connecting to the database and before the Page_Load event. Note: This is a global function. |
Page_Unloaded |
This event will be called by all PHP pages at the end of the page. If the pages involves database connection, it will be called before closing database connection and after the Page_UnLoad event. Note: This is a global function. |
Global Code |
Code to be included in all PHP pages. This may contain your constants, variables and functions. |
User_CustomValidate |
For use with security. (See Security Settings) This event is fired before default user validation. You can use this event to validate the user yourself. The arguments are the user name and password the user entered. Note: This event is a security class member.
Note: This event is not fired for the hard-coded administrator.
Example
Login user by Windows user name. Note that in this simple example, Advanced Security is not used so you can return TRUE to skip default validation. If you use Advanced Security, you still need the user information such as User ID and User Level. The user table is still required to store user information, although the password field is unnecessary. And you need to return FALSE to continue with default validation.
// Note: This event is a Security class member, so you can refer to other members of the Security class directly
function User_CustomValidate(&$usr, &$pwd) {
// e.g. Simple Windows authentication
if (ew_ServerVar("LOGON_USER") <> "") {
$usr = ew_ServerVar("LOGON_USER");
return TRUE; // In this example, no Advanced Security, skip default validation
}
return FALSE; // Return FALSE to continue with default validation after event exits,
} |
User_Validated |
For use with security. (See Security Settings) This event is fired after successful user login. The user info is passed to the event as an array (see note), you can get more info of the user and use it as you need. Note: This event is a security class member.
Notes:
- This event is not fired for the hard-coded administrator.
- The argument is an array, you can get a field vaue by $rs['<fieldname>'].
Example
Add current user's country to session variable
// Note: This event is a Security class member, so you can refer to other members of the Security class directly
function User_Validated(&$rs) {
$_SESSION["UserCountry"] = $rs['Country'];
} |
UserLevel_Loaded |
For use with User Level security. (See Security Settings) This event is fired after successful user login and after the User Level settings are loaded. It is possible to do actions such as changing or adding User Level permissions. Note: This event is a security class member.
Example
Change the permissions of an User Level for a table
// Note: This event is a Security class member, so you can refer to other members of the Security class directly
function UserLevel_Loaded() {
$this->DeleteUserPermission("Sales", "orders", EW_ALLOW_ADD);
$this->AddUserPermission("Sales", "orders", EW_ALLOW_EDIT);
} |
UserID_Loading |
For use with User ID security. (See Security Settings) This event is fired after successful user login and before loading the User ID and its child User IDs of the current user. These User IDs determine which records the current user can access. It is possible to do actions such as changing the User ID of the current user so the user can access records that he/she can access by its original User ID. Note: This event is a security class member.
Example
Change the user's User ID to his parent user's user ID and let the user access more records (accessible by the parent user).
// Note: This event is a Security class member, so you can refer to other members of the Security class directly
function UserID_Loading() {
if (CurrentParentUserID() <> "") {
$this->CurrentUserID = CurrentParentUserID();
}
} |
UserID_Loaded |
For use with User ID security. (See Security Settings) This event is fired after loading the User ID and its child User IDs of the current user. These User IDs determine which records the current user can access. It is possible to do actions such as adding or deleting the loaded User IDs for the current user so the user can access more or less records that he/she can access by its originally loaded User IDs. Note: This event is a security class member.
Example
Add more User IDs to the user and let the user access more records
// Note: This event is a Security class member, so you can refer to other members of the Security class directly
function UserID_Loaded() {
if (CurrentUserName() == "nancy") {
$this->AddUserID($this->GetUserIDByUserName("janet"));
}
} |
Menu_Rendering |
This event is fired before the menu is rendered. You can manipulate the menu items in this event. The argument is the menu object. (See Menu Object belwo.) Note: This is a global function.
Example 1
Add an additional menu item to the menu for logged in users only.
function Menu_Rendering(&$Menu) {
if ($Menu->IsRoot) { // Root menu
$Menu->AddMenuItem(10000, "MyMenuText", "MyPage.php", -1, "", IsLoggedIn());
}
}
Example 2
Remove all the default menu items and use your own menu items.
function Menu_Rendering(&$Menu) {
if ($Menu->IsRoot) { // Root menu
$Menu->Clear();
$Menu->AddMenuItem(1, "MyMenuText1", "MyPage1.php", -1);
$Menu->AddMenuItem(2, "MyMenuText2", "MyPage2.php", -1);
}
}
|
MenuItem_Adding |
This event is fired for custom menu items before it is added to the menu. The menu item info is passed to the event as an instance of the crMenuItem object, refer to the generated ewrfn*.php for members of the object. Return False if you don't want to show the menu item. Note: This is a global function.
Example
Only show a menu item after the user has logged in
function MenuItem_Adding(&$Item) {
//var_dump($Item);
// Return False if menu item not allowed
global $Security;
if ($Item->Text == "Download") {
return $Security->IsLoggedIn();
} else {
return TRUE;
}
}
Note: If you integrate with PHPMaker, since the menu items are imported to the PHPMaker project, you should use the MenuItem_Adding server event in the PHPMaker project.
|
Table-Specific -> Report Page |
Page_Load |
This event will be called after connecting to the database. |
Page_Unload |
This event will be called before closing database connection. |
Filters_Load |
This event allows you to add custom filter to the popup filter of the fields. (Previously named as CustomFilter_Load.)
Note: This event is a table class member, so you can refer to the fields using $this-><field>.
Example
Add 'StartsWithA' filter to a string field. You can use the ewrpt_RegisterCustomFilter() function to add your custom filter, the parameters of the function are:
- fld - the field object
- FilterName - the filter name
- DisplayName - the display name of the filter to be displayed in the popup filter
- FunctionName - the PHP function to be called for building the WHERE clause. The function must have one and only one parameter which is the field expression to be used in the WHERE clause. You can put the function in Global Code. (Note: The following example function is already in the template, do not put it in the Global Code again.)
function GetStartsWithAFilter($FldExpression) {
return $FldExpression . " LIKE 'A%'";
}
Then you can enter event code as below:
function Filters_Load() {
ewrpt_RegisterCustomFilter($this->MyStringField, 'StartsWithA', 'Starts With A', 'GetStartsWithAFilter');
ewrpt_UnregisterCustomFilter($this->MyDateField, 'LastTwoWeeks');
}
|
Page_FilterValidated |
This event will be called after the search criteria is assigned to the field objects. You can modify the search criteria in this event.
This event is a member of the table class. There is no arguments for this event. To change the search criteria, change the SearchValue, SearchOperator, SearchCondition, SearchValue2, SearchOperator2 property of the field object. |
Page_DataRendering |
This event will be called before report rendering. You can use this event to add HTML above the report. The parameter is:
header - set your HTML to this parameter.
Note: This event is a page class member. |
Page_DataRendered |
This event will be called after report rendering. You can use this event to add HTML below the report. The parameter is:
footer - set your HTML to this parameter.
Note: This event is a page class member. |
Row_Rendering |
This event will be called before rendering (e.g. applying the View Tag settings) a record. Note: This event is a table class member. |
Row_Rendered |
This event will be called after rendering a record.
This is an extremely useful event for conditional formatting, you can do a lot of things with this event, such as changing font color, font styles, row background color, cell background color, etc. by changing the table or field class properties in the event according to field values.
The table class has a RowAttrs property which is an associative array of HTML attributes for the table row. The field class has CellAttrs, ViewAttrs for the table cell and View Tag of the field respectively. The keys of these arrays must be valid HTML attributes for the HTML tag, always use lowercase for the keys. The attribute values will be outputted as double quoted attributes, so if you have double quotes in your values, try to use single quotes if possible, or use """.
Note: This event is a table class member, so you can refer to the fields using $this-><field>.
To view the properties of the field class for development or debugging, you can use the PHP's var_dump function in the server event, e.g.
var_dump($this->RowAttrs);
var_dump($this->Trademark);
var_dump($this->Trademark->CellAttrs);
var_dump($this->Trademark->ViewAttrs);
Example
Click "Cars" node on the
database panel, select [Server Events/Client Scripts], and click [Table
Specific] - > [Common] - > [Row_Rendered] server event. The default Row_Rendered event code is shown. Change as follow:
function Row_Rendered() {
// Change the row color
if ($this->Trademark->ViewValue == "BMW") {
$this->RowAttrs["style"] = "color: red; background-color: #ccffcc";
}
// Change the cell color
if ($this->Cyl->CurrentValue == 4) {
$this->Cyl->CellAttrs["style"] = "background-color: #ffcccc";
} elseif ($this->Cyl->CurrentValue == 6) {
$this->Cyl->CellAttrs["style"] = "background-color: #ffcc99";
} elseif ($this->Cyl->CurrentValue == 8) {
$this->Cyl->CellAttrs["style"] = "background-color: #ffccff";
}
// Change text style
if ($this->Category->CurrentValue == "SPORTS") {
$this->Category->ViewAttrs["style"] = "color: #00cc00; font-weight: bold; background-color: #ffff99";
}
}
|
Cell_Rendered |
This event will be called after rendering a cell.
Note: This event is fired before Row_Rendered event, if you set the same setting in the Row_Rendered event, it will be overwritten by the Row_Rendered event.
This is another extremely useful event for conditional formatting. It is especially useful for the dynamic columns for Crosstab reports as there are many dynamic columns for the same Column Field in the report. This event saves you from accessing the arrays for the columns, you can get/set the values passed to the event as arguments directly. The arguments are:
$Field - the field object
$CurrentValue - current value of the cell (unformatted)
$ViewValue - view value of the cell (formatted)
$ViewAttrs - assocative array of the View Tag attributes
$CellAttrs - assocative array of the table cell attributes
$HrefValue - href attribute if the hyperlink for the view value of the cell
The keys of the $CellAttrs and $ViewAttrs arrays must be valid HTML attributes for the HTML tag, always use lowercase for the keys. The attribute values will be outputted as double quoted attributes, so if you have double quotes in your values, try to use single quotes if possible, or use """.
Note: This event is a table class member, so you can refer to the fields using $this-><field>.
Example
Click "Cars" node on the
database panel, select [Server Events/Client Scripts], and click [Table
Specific] - > [Common] - > [Row_Rendered] server event. The default Row_Rendered event code is shown. Change as follow:
function Cell_Rendered(&$Field, $CurrentValue, &$ViewValue, &$ViewAttrs, &$CellAttrs, &$HrefValue) {
// Change the cell color for the Cyl field
if ($Field->FldName == "Cyl") {
if ($CurrentValue == 4) {
$CellAttrs["style"] = "background-color: #ffcccc";
} elseif ($CurrentValue == 6) {
$CellAttrs["style"] = "background-color: #ffcc99";
} elseif ($CurrentValue == 8) {
$CellAttrs["style"] = "background-color: #ffccff";
}
}
}
|
Chart_Rendering |
This event is fired before the chart XML is built. You can still change the chart properties using this event, the parameters is:
chart - the chart object instance which contain all the information about the chart to be rendered. It is an instance of the crChart class, refer to ewrfn*.php for members of the object. |
Chart_Rendered |
This event is fired after the chart XML is built and before the XML is outputted. You can still modify the XML using this event, the parameters is:
chart - the chart object instance which contain all the information about the chart to be rendered. It is an instance of the crChart class, refer to ewrfn*.php for members of the object. This parameter is read-only. You can get the XML document by $chart->XmlDoc and manipulate it by by PHP DOM functions, then save it back to the $chartxml for output. Refer to Chart XML API for chart properties.
chartxml - the XML (string) to be outputted. You can directly manipulate it as string by string functions.
Example
Set chart subcaption by code
function Chart_Rendered($chart, &$chartxml) {
$doc = $chart->XmlDoc; // Get the DOMDocument object
$doc->documentElement->setAttribute('subCaption', 'My SubCaption');
$chartxml = $doc->saveXML(); // Output the XML
}
|
Email_Sending |
This event is fired before the email is sent. You can customize the email content using this event. Email_Sending event has the following parameters:
Email - the email object instance which contain all the information about the email to be sent. It is an instance of the crEmail class, refer to ewrfn*.php for members of the object.
Args - an array which contains additional information. By default it is empty.
Return False in the event if you want to cancel the email sending.
Note: This event is a table class member.
Example
Use a field value of the the new record in the email
function Email_Sending(&$Email, &$Args) {
//var_dump($Email);
//exit();
global $Page;
$Email->Content .= "\nSent by " . CurrentUserName();
return TRUE;
}
|
Message_Showing |
Use this event to change the error message to be displayed in the page. |
Form_CustomValidate |
This event is fired after the normal form validation. You can use this event to do your own custom validation. In general, the form data can be accessed by $<Table>-><Field>->FormValue (e.g. $Cars->HP->FormValue). Refer to the generated code for actual variable names.
A parameter CustomError is passed to the event, add your error message and return False if the form values do not pass your validation.
Note: This event is a page class member.
Example
Make sure an integer field value meet a certain requirement
function Form_CustomValidate(&$CustomError) {
global $orders;
if (intval($orders->Qty->FormValue) % 10 <> 0) {
// Return error message in CustomError
$CustomError = "Quantity must be multiples of 10.";
return FALSE;
} else {
return TRUE;
}
} |
Other -> Login Page |
Page_Load |
This event will be called at the beginning of the page. |
Page_Unload |
This event will be called at the end of the page. |
Message_Showing |
Use this event to change the error message to be displayed in the page. |
Form_CustomValidate |
This event is fired after the normal form validation. You can use this event to do your own custom validation.
A parameter CustomError is passed to the event, add your error message and return False if the form values do not pass your validation.
Note: This event is a page class member. |
Other -> Logout Page |
Page_Load |
This event will be called at the beginning of the page. |
Page_Unload |
This event will be called at the end of the page. |
Client Scripts
In general, each page has two blocks of JavaScripts:
Client Script - the first block of JavaScript to be included at the beginning of the page, you can put your JavaScript variables and functions there. The View Tag (for display) and Edit Tag (for input) of the fields supports Custom Attributes (See Field Setup) so you can add your own attributes to work with your own JavaScript included here.
Startup Script - the second block of JavaScript to be included at the end of the page, you can put code here to "start up" your JavaScript.
Global -> Pages with header/footer |
Client Script |
The script will be placed in the header and therefore included in all pages with header. |
Startup Script |
The script will be placed in the footer and therefore included in all pages with footer. |
Global Code |
JavaScript code to be included in all pages with header. This may contain your global constants, variables and functions. |
Table-Specific -> Report Page |
Client Script |
The script will be placed after the header. |
Startup Script |
The script will be placed before the footer. |
Form_CustomValidate |
This function is called after the normal form validation. You can use this event to do your own custom validation. The form object can be accessed by the parameter fobj. In general, the field can be referred to as fobj.elements["x_FieldName"]. (The element names have different names in Inline/Grid Add/Edit of List page, always refer to the generated code for the actual form element names.) Return False if the form values do not pass your validation.
Note: This function is a member of the JavaScript page class.
Example
Make sure an integer field value meet a certain requirement
function(fobj) { // DO NOT CHANGE THIS LINE!
var qty = parseInt(fobj.elements["x_Qty"].value);
if (qty % 10 != 0) {
return ew_OnError(this, fobj.elements["x_Qty"], "Order quantity must be multiples of 10."); // return false if invalid
}
return true;
} |
Chart_Rendering |
This event is fired when the JavaScript chart object has finished loading the XML data and before rendering.
The event arguments are :
eventObject - This object contains eventId, eventType and sender properties. You can check the chart id (i.e. eventObject.sender.id) to identify the chart.
argumentsObject - This is passed as a blank object. |
Chart_Rendered |
This event is raised when the JavaScript chart object has finished rendering. This call is made only once per loaded chart SWF (even if new data is supplied to it). It can be used to invoke any further JavaScript methods on chart.
The event arguments are :
eventObject - This object contains eventId, eventType and sender properties. You can check the chart id (i.e. eventObject.sender.id) to identify the chart.
argumentsObject - This is passed as a blank object. |
Other -> Login Page |
Client Script |
The script will be placed after the header. |
Startup Script |
The script will be placed before the footer. |
Form_CustomValidate |
This function is called after the normal form validation. You can use this event to do your own custom validation. The form object can be accessed by the parameter fobj. Return False if the form values do not pass your validation. |
Note: It is recommended that you develop your server event and client scripts in the generated script so you can edit and test it immediately. When you finish your custom script, copy it to PHP Report Maker custom script editor and save it.
Objects in PHP Report Maker Generated
Code
The following
objects are available in the generated code and you can use them in the
Server Events to add more power to the code. The most important objects are:
Page Object
The Page object is generated for most pages. You can access the
object properties using the -> notation (e.g. $Page->PageID). The methods and properties of the page class varies with page, for
the complete list of methods and properties, please refer to the generated class
"cr<Table>_<PageID>" (e.g. crCars_rpt) in the generated scripts.
Table Object
A cr<Table> Class is generated for each table and the
<Table> object is instantiated for all table level pages. For example,
the "crCars" class is generated for the "Cars" table
and is instantiated as the "Cars" object. You can access the
object properties using the -> notation (e.g. $Cars->CurrentAction). For
the complete list of methods and properties, please refer to the class
"cr<Table>" in the generated file.
Field Object
A <Field> object is generated for each field in a
table. For example, the "Trademark" object is generated for
the "Trademark" field in the "Cars" table. You can
access the object properties using the -> notation (e.g. $Cars->Trademark->CurrentValue).
For the complete list of methods and properties, please refer to the class
"crField" in the generated file "ewrfn*.php".
Chart Object
A <Chart> object is generated for each chart in a
table. For example, the "Chart1" object is generated for
the chart named "Chart1" for the table. You can
access the object properties using the -> notation (e.g. $report1->Chart1).
For the complete list of methods and properties, please refer to the class
"crChart" in the generated file "ewrfn*.php".
Security Object
The Security object is used to store the current Advanced Security settings. Please refer to the class "crAdvancedSecurity" in the generated
file "ewrfn*.php" for the complete list of methods and properties.
Email Object
The Email object contains the required information of the email to be sent, the instance of the object will be passed to the Email_Sending events as argument and let you modify the email. Please refer to the class "crEmail" in the generated
file "ewrfn*.php" for the complete list of methods and properties.
Menu Object
The Menu object contains all information of a menu, the instance of the menu will be passed to the Menu_Rendering events as argument and let you work with the menu. Please refer to the class "crMenu" in the generated
file "ewrfn*.php" for the complete list of methods and properties.
MenuItem Object
The MenuItem object contains all information of the menu item, the instance of the menu item will be passed to the MenuItem_Adding events as argument and let you work with the menu item. Please refer to the class "crMenuItem" in the generated
file "ewrfn*.php" for the complete list of methods and properties.
ListOpions Object
The ListOptions object contains all information of the export links in the report page. Please refer to the class "crListOptions" in the generated
file "ewrfn*.php" for the complete list of methods and properties.
Language Object
The language Object lets you retrieve a phrase of the active language during runtime. The phrase can be retrieved in the generated scripts using methods such as Phrase, TablePhrase and FieldPhrase. Please refer to the class "crLanguage" in the generated
file "ewrfn*.php" for the complete list of methods and properties.
There are a few other objects in the generated code, please refer to the source code of the
file "ewrfn*.php" in the generated scripts.
Some Global Functions
The following
are some useful global function available in the generated code for you to get information easier in server events:
Function name |
Arguments |
Description |
Example |
G |
varname |
Get a global variable. |
$security = G("Security"); // get the global Security object |
CurrentUserName |
- |
Get current user name. |
$username = CurrentUserName(); |
CurrentUserID |
- |
For used with User ID Security (see Security Settings). Get current User ID. |
$userid = CurrentUserID(); |
CurrentUserLevel |
- |
For used with User Level Security (see Security Settings). Get current user's User Level ID (integer). |
$levelid = CurrentUserLevel(); |
CurrentUserInfo |
fieldname |
For used with Advanced Security (see Security Settings). Get current user's info from the user table. The argument is the field name in the user table. |
$email = CurrentUserInfo("email"); |
CurrentPageID |
- |
Get current page ID. A page ID identifies the page type, it can be "rpt", "summary", "crosstab", etc. |
if (CurrentPageID() == "crosstab") {
...your code...
} |
CurrentPage |
- |
Get current page object. |
$page = CurrentPage(); |
CurrentTable |
- |
Get current table object. |
$table = CurrentTable(); |
IsLoggedIn |
- |
For used with Advanced Security (see Security Settings). Get the login status of the current user. |
if (IsLoggedIn()) {
...your code...
} |
There are many other functions in the generated code, please refer to the source code of the
file "ewrfn*.php" in template or generated scripts.
|